Core Concepts Covered
- Use the built-in android.R.layout.simple_list_item_1 to create an Android UI List.
- Use android.widget.ArrayAdapter to display a List of Strings data in the Android UI List.
- Create class extending android.app.ListActivity.
- Obtain data in List of Strings data structure.
- Create an android.widget.ArrayAdapter that connects the List of Strings data to android.R.layout.simple_list_item_1.
- Bind the android.widget.ListAdapter using the ListActivity.setListAdapter method.
1. Create class extending android.app.ListActivity.
public class MainActivity extends ListActivity {
@Override
protected final void onCreate(final Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
}
For this tutorial, we will use a List of Strings containing the 13 US original colonies. View the full UsState.java code listing.
public final class UsState {
public static final List<String> ORIGINAL_COLONIES = initColonies();
private UsState() { }
private static List<String> initColonies() {
final List<String> colonies = new ArrayList<String>();
colonies.add("Delaware");
colonies.add("Pennsylvania");
colonies.add("New Jersey");
return Collections.unmodifiableList(colonies);
}
}
private ListAdapter createListAdapter(final List<String> list) {
return new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, list);
}
4. Bind the android.widget.ListAdapter using the ListActivity.setListAdapter method.
public class MainActivity extends ListActivity {
@Override
protected final void onCreate(final Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
final ListAdapter listAdapter = createListAdapter(UsState.ORIGINAL_COLONIES);
setListAdapter(listAdapter);
}
}
The final result will look like this
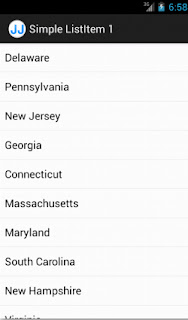
Source Code: Git | SVN | Download Zip
smm panel
ReplyDeleteSmm panel
iş ilanları
instagram takipçi satın al
hirdavatciburada.com
beyazesyateknikservisi.com.tr
SERVİS
tiktok jeton hilesi
üsküdar alarko carrier klima servisi
ReplyDeletependik toshiba klima servisi
pendik beko klima servisi
çekmeköy toshiba klima servisi
çekmeköy beko klima servisi
ataşehir beko klima servisi
maltepe lg klima servisi
kadıköy lg klima servisi
maltepe alarko carrier klima servisi